Image Processing #9 - Image Splitting
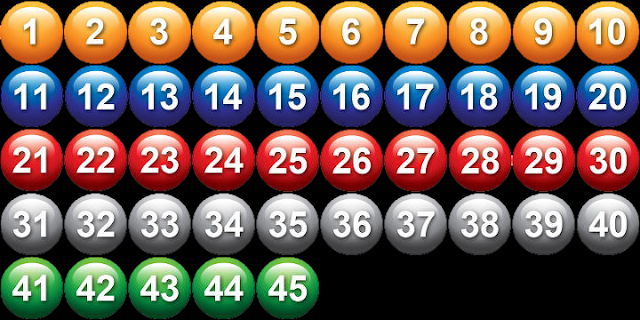
Recently, I had to separate a image containing multiple balls. In the case of one or two balls, you can use an image editing program to separate them, but if the number of images increases, it can be much faster to create a simple program to separate them. <lotto.png> If you use numpy's hsplit and vsplit functions, you can separate the images into N by M pieces. You can separate lottery balls into 1 ~ 45.png files with the following simple code. #-*- coding:utf-8 -*- import numpy as np import cv2 # split into 10 X 5 H_Count = 10 V_Count = 5 file = './lotto.png' img = cv2 . imread(file, cv2 . IMREAD_COLOR) height, width, channels = img . shape count = 1 h_img = np . vsplit(img, V_Count) for i in h_img: j = np . hsplit(i, H_Count) for k in j: name = "%d.png" % (count) cv2 . imwrite(name, k) count += 1 <img_split.py> <splitted image files>